Grouped table view is an extension of the normal table view in iPhone. It displayes the data in several sectioned lists. Each section has a header and number of rows associated with it. Examples of grouped table are the ‘contacts’ list, a dictionary,etc. In this tutorial, we will create a grouped table using a dictionary, to create a names list,unlike the plain table in which we had used an array. So lets get started!
Step 1: Create a view based application and name it ‘GroupedTable’.
Step 2: Put the following code in ‘GroupedTableViewController.h’
03 | @interface GroupedTableViewController : UIViewController { |
04 | NSDictionary *tableContents; |
08 | @property ( nonatomic ,retain) NSDictionary *tableContents; |
09 | @property ( nonatomic ,retain) NSArray *sortedKeys; |
Here, we have declared a dictionary to hold the section headers and their respective row contents. And an array to hold the sorted keys of the dictionary.
Step 3: Now, open ‘GroupedTableViewController.m’ file and put the following code in it.
001 | #import "GroupedTableViewController.h" |
003 | @implementation GroupedTableViewController |
004 | @synthesize tableContents; |
005 | @synthesize sortedKeys; |
009 | NSArray *arrTemp1 = [[ NSArray alloc] |
010 | initWithObjects: @"Andrew" , @"Aubrey" , @"Alice" , nil ]; |
011 | NSArray *arrTemp2 = [[ NSArray alloc] |
012 | initWithObjects: @"Bob" , @"Bill" , @"Bianca" , nil ]; |
013 | NSArray *arrTemp3 = [[ NSArray alloc] |
014 | initWithObjects: @"Candice" , @"Clint" , @"Chris" , nil ]; |
015 | NSDictionary *temp =[[ NSDictionary alloc] |
016 | initWithObjectsAndKeys:arrTemp1, @"A" ,arrTemp2, |
017 | @"B" ,arrTemp3, @"C" , nil ]; |
018 | self .tableContents =temp; |
020 | self .sortedKeys =[[ self .tableContents allKeys] |
021 | sortedArrayUsingSelector: @selector (compare:)]; |
029 | [tableContents release]; |
030 | [sortedKeys release]; |
034 | #pragma mark Table Methods |
036 | - ( NSInteger )numberOfSectionsInTableView:(UITableView *)tableView{ |
037 | return [ self .sortedKeys count]; |
040 | - ( NSString *)tableView:(UITableView *)tableView |
041 | titleForHeaderInSection:( NSInteger )section |
043 | return [ self .sortedKeys objectAtIndex:section]; |
046 | - ( NSInteger )tableView:(UITableView *)table |
047 | numberOfRowsInSection:( NSInteger )section { |
048 | NSArray *listData =[ self .tableContents objectForKey: |
049 | [ self .sortedKeys objectAtIndex:section]]; |
050 | return [listData count]; |
053 | - (UITableViewCell *)tableView:(UITableView *)tableView |
054 | cellForRowAtIndexPath:( NSIndexPath *)indexPath { |
055 | static NSString *SimpleTableIdentifier = @"SimpleTableIdentifier" ; |
057 | NSArray *listData =[ self .tableContents objectForKey: |
058 | [ self .sortedKeys objectAtIndex:[indexPath section]]]; |
060 | UITableViewCell * cell = [tableView |
061 | dequeueReusableCellWithIdentifier: SimpleTableIdentifier]; |
065 | cell = [[[UITableViewCell alloc] |
066 | initWithStyle:UITableViewCellStyleDefault |
067 | reuseIdentifier:SimpleTableIdentifier] autorelease]; |
075 | NSUInteger row = [indexPath row]; |
076 | cell.textLabel.text = [listData objectAtIndex:row]; |
081 | - ( void )tableView:(UITableView *)tableView |
082 | didSelectRowAtIndexPath:( NSIndexPath *)indexPath { |
083 | NSArray *listData =[ self .tableContents objectForKey: |
084 | [ self .sortedKeys objectAtIndex:[indexPath section]]]; |
085 | NSUInteger row = [indexPath row]; |
086 | NSString *rowValue = [listData objectAtIndex:row]; |
088 | NSString *message = [[ NSString alloc] initWithFormat:rowValue]; |
089 | UIAlertView *alert = [[UIAlertView alloc] |
090 | initWithTitle: @"You selected" |
091 | message:message delegate: nil |
092 | cancelButtonTitle: @"OK" |
093 | otherButtonTitles: nil ]; |
097 | [tableView deselectRowAtIndexPath:indexPath animated: YES ]; |
viewdidLoad: Here, we populate the dictionary using three arrays of names as objects and alphabets as keys. Then we sort the array ‘allKeys’ of the dictionary and put it in sortedKeys.
Table Methods
numberOfSectionsInTableView : Returns the count of keys in the dictionary.
titleForHeaderInSection : Returns the value of key for that particular section.
cellForRowAtIndexPath : Here, listdata is an array which is assigned the value for the key corresponding to the respective section. For every section, the contents of listdata are displayed.
didSelectRowAtIndexPath : In this method, we display an alert view when a row is selected.
Step 4 : Open the ‘GroupedTableViewController.xib’. Drag and drop a table view on it. Select the table view and open its Attributes Inspector (command+1). Change the table style from ‘Plain’ to ‘Grouped’.
Step 5 : Open the Connections Inspector (command+2) for the table and link its datasource and delegate to the file’s owner.
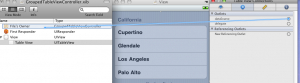
Step 6 : You can download the source code here.Save,build and run the project. The output will be as follows:
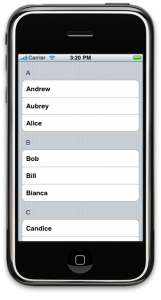
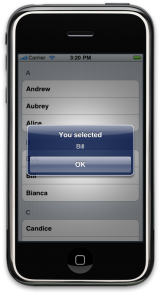
Không có nhận xét nào:
Đăng nhận xét